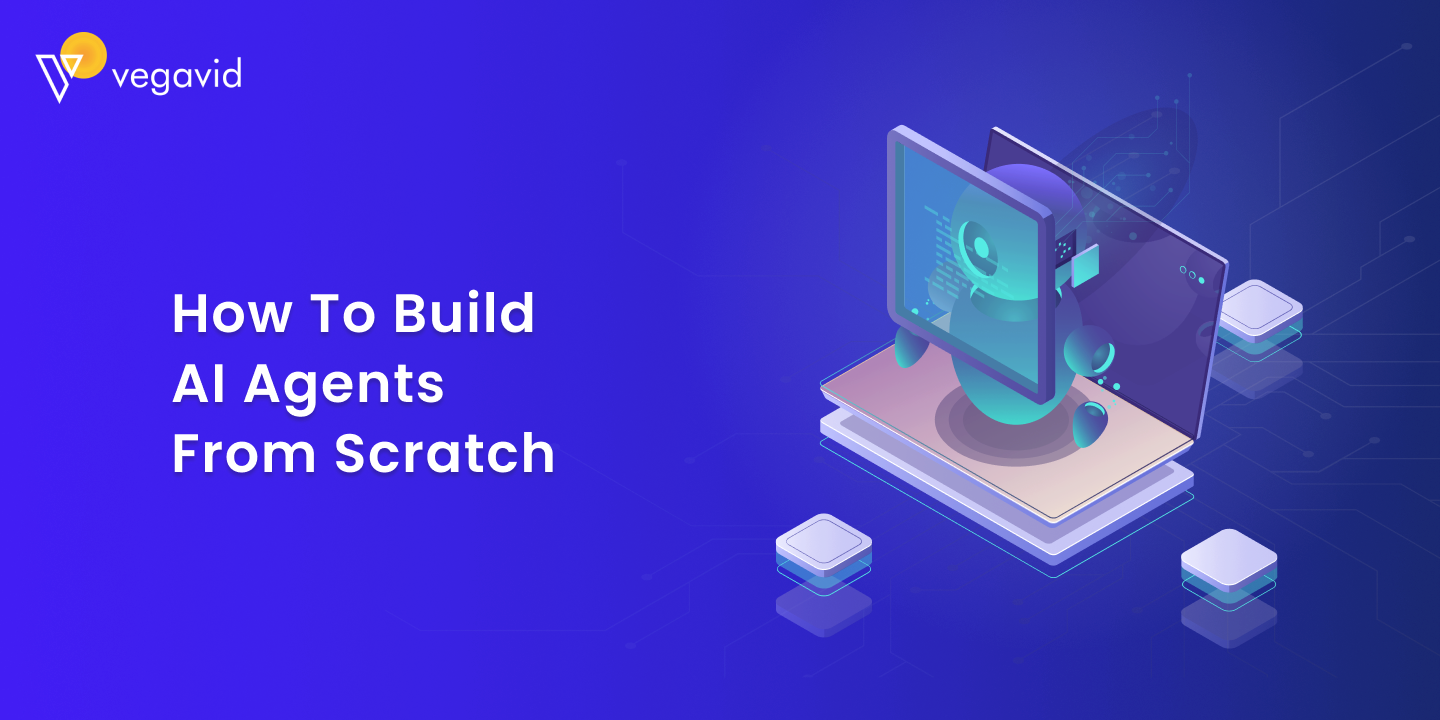
The world of AI agent development is increasingly accessible thanks to the power of open-source tools. These freely available resources not only lower the barrier to entry but also foster a collaborative environment where developers can learn, share, and improve together. Choosing the right tools is the first crucial step in your AI agent development journey.
Python is the undisputed king of AI programming. Its readability, extensive libraries, and large community support make it the ideal language for beginners and experts alike. For building AI agents, several powerful Python libraries stand out:
- TensorFlow: Developed by Google, TensorFlow is a robust library for numerical computation and large-scale machine learning. It’s particularly well-suited for deep learning, a technique often used in creating sophisticated AI agents.
- PyTorch: A strong competitor to TensorFlow, PyTorch is known for its dynamic computation graph and ease of use, especially for research and rapid prototyping. It’s also gaining popularity in building AI agents.
- scikit-learn: This library provides a wide range of machine learning algorithms, tools for model selection, and evaluation metrics. While not specifically designed for AI agents, it’s invaluable for tasks like classification, regression, and clustering, which are often components of agent systems.
Beyond these core libraries, you’ll also find helpful tools for specific agent functionalities. For example, libraries for natural language processing (NLP) can be crucial for building agents that interact with text.
To get started without any upfront costs, consider leveraging free cloud platforms with free tiers.
- Google Colab: This cloud-based Jupyter notebook environment offers free access to GPUs, making it ideal for experimenting with computationally intensive machine learning tasks.
- Kaggle: While known primarily for data science competitions, Kaggle also provides a free computing environment and datasets, offering a great platform to learn and practice.
While these tools offer incredible power and flexibility, it’s important to be aware of their limitations. Some libraries might have a steeper learning curve than others. Free cloud platforms might have usage limits. However, for beginners, these tools provide an excellent starting point for exploring the exciting world of AI agent development without any financial investment.
Setting Up Your Development Environment
Now that you’ve chosen your tools, it’s time to set up your development environment. We’ll focus on installing Python and the key libraries mentioned earlier. Since Python is essential, we’ll begin there.
1. Installing Python:
- Windows: Download the latest version of Python from https://www.python.org/downloads/. During installation, make sure to check the box that says “Add Python to PATH.” This will allow you to run Python commands from your command prompt or PowerShell.
- macOS: Python might already be pre-installed. You can check by opening Terminal and typing
python3 --version
. If it’s not installed or you want a newer version, you can use a package manager like Homebrew (brew install python@3.9
– replace 3.9 with your desired version) or download from the Python website. - Linux: Most Linux distributions come with Python pre-installed. You can check the version using
python3 --version
. If needed, you can install or update using your distribution’s package manager (e.g.,apt-get install python3
on Debian/Ubuntu,yum install python3
on CentOS/RHEL).
2. Creating a Virtual Environment (Highly Recommended):
Virtual environments isolate your project dependencies, preventing conflicts between different projects.
- Open your command prompt or terminal.
- Navigate to your project directory (where you want to create your AI agent project).
- Create a virtual environment:
python3 -m venv my_ai_env
(replacemy_ai_env
with your desired environment name). - Activate the environment:
- Windows:
my_ai_env\Scripts\activate
- macOS/Linux:
source my_ai_env/bin/activate
- Windows:
You’ll see the environment name in parentheses in your terminal, indicating it’s active.
3. Installing Libraries:
Now, with your virtual environment activated, you can install the necessary libraries using pip, Python’s package installer:
pip install tensorflow
pip install torch
pip install scikit-learn
For specific versions or GPU support, refer to the official documentation for each library:
- TensorFlow: https://www.tensorflow.org/install
- PyTorch: https://pytorch.org/get-started/locally/
- scikit-learn: https://scikit-learn.org/stable/install.html
4. Setting up Google Colab (Optional):
If you’re using Google Colab, you don’t need to install anything locally. Just go to https://colab.research.google.com/ and create a new notebook. Colab comes pre-installed with many popular libraries, but you can install additional ones using pip in the notebook cells (e.g., !pip install tensorflow
).
5. Setting up Kaggle (Optional):
Similar to Colab, Kaggle provides a ready-to-use environment. When you create a new notebook on Kaggle, the necessary libraries are usually already included.
By following these steps, you’ll have a fully functional development environment ready for building your free AI agents. Remember to always activate your virtual environment before working on your project to keep your dependencies organized.
Fundamental of AI Agents
AI agents are designed to mimic intelligent behavior. They achieve this through a combination of key components that work together seamlessly. Understanding these core concepts is fundamental to building effective AI agents.
- Perception: This is how the agent “sees” the world. It involves gathering information from the environment through sensors. Think of it like our senses of sight, hearing, and touch. A camera acts as a sensor for a self-driving car, while a microphone acts as a sensor for a voice assistant. The perception module then processes this raw data into a usable format.
- Reasoning: This is the “thinking” part. The agent uses the perceived information, combined with its internal knowledge, to make decisions and plan actions. It’s like our brain processing sensory input and deciding what to do. A chess-playing AI uses reasoning to evaluate possible moves and choose the best one.
- Action: This is how the agent interacts with the world. It involves executing the actions chosen by the reasoning module. It’s like our muscles carrying out our decisions. A robot arm performing a task or a chatbot sending a message are examples of actions.
- Learning (Optional): This is the ability to improve performance over time. The agent analyzes its past experiences and adjusts its strategies accordingly. It’s like us learning from our mistakes. A spam filter learning to identify new spam emails is an example of a learning agent.
How to Choose the Right Type of AI Agent?
Different AI agents are designed for different tasks. Here are some common types:
- Reflex Agents: These are the simplest agents. They react directly to perceptions based on pre-defined rules. Think of a thermostat: it reacts to temperature changes by turning the heating or cooling on or off. These are easy to implement but lack flexibility. A good starting point for a first project if you want to focus on the perception-action loop.
- Goal-Based Agents: These agents have a specific goal they want to achieve and plan a sequence of actions to reach it. Think of a navigation app finding the best route to your destination. Suitable if your project has a clear objective.
- Utility-Based Agents: These agents go a step further by assigning a “utility” or value to different outcomes. They choose actions that maximize their overall utility. Think of a game-playing AI choosing moves that give it the highest chance of winning. More complex but offer greater flexibility.
- Learning Agents: These agents can learn and improve their performance over time. Think of a recommendation system that learns your preferences. These are more advanced and might be better for later projects once you’ve grasped the basics.
For your first AI agent project, starting with a reflex agent is highly recommended. It allows you to focus on the fundamental concepts of perception and action without the added complexity of planning or learning. Once you’ve mastered this, you can move on to more sophisticated agent types as you gain experience.
Step-by-Step Tutorial: How To Build A Simple AI Agent For Free?
Let’s create a basic reflex agent in Python that simulates a light switch. This agent will perceive the state of a “room” (light on or off) and react accordingly.
Step 1: Setting up the Environment
Make sure you have Python installed and a virtual environment activated (as described in the previous section).
Step 2: Defining the Perception
Our agent’s perception will be represented by a simple string: “on” or “off.”Python
room_state = "off" # Initial state of the room
Step 3: Defining the Rules (Reflexes)
We’ll use a dictionary to store our rules. The keys will be the perceived states, and the values will be the corresponding actions.Python
rules = {
"off": "turn on",
"on": "turn off"
}
Step 4: Implementing the Agent’s Logic
def light_switch_agent(room_state):
action = rules.get(room_state) # Look up the action based on the current state
if action: # If rule exists for current state
print(f"The room is {room_state}. Agent action: {action}")
return action
else:
print(f"The room is {room_state}. No rule defined for this state")
return "no_action"
Step 5: Running the Agent
room_state = "off"
light_switch_agent(room_state) # First execution
room_state = "on"
light_switch_agent(room_state) # Second execution
room_state = "dim" # Test with an undefined room state
light_switch_agent(room_state) # Third execution
Debugging Tips and Common Errors:
- Indentation Errors: Python relies heavily on indentation. Make sure your code blocks are correctly indented.
- KeyError: If you try to access a key that doesn’t exist in the
rules
dictionary, you’ll get aKeyError
. Use the.get()
method (as shown above) to handle this gracefully. - Type Errors: Double-check that your variables are of the correct type (e.g., strings for the room state).
Example Project Ideas
Now that you’ve built a simple reflex agent, here are some ideas for expanding your skills:
- Simple Chatbot: Create a chatbot that responds to basic user input based on pre-defined rules. You can start with simple greetings and responses, then add more complex interactions. Consider using NLP libraries for more advanced chatbot features.
- Basic Game-Playing Agent: Develop an agent that can play a simple game like Tic-Tac-Toe. You can use search algorithms to explore possible moves and choose the best one.
- File Organizer Agent: Build an agent that automatically categorizes files based on their type or content. This can involve using regular expressions or machine learning techniques.
Resources:
- Python Tutorials: https://www.python.org/about/gettingstarted/
- TensorFlow Tutorials: https://www.tensorflow.org/tutorials
- PyTorch Tutorials: https://pytorch.org/tutorials/
- scikit-learn Tutorials: https://scikit-learn.org/stable/tutorial/index.html
These resources will provide you with a solid foundation for building more complex AI agents. Remember to practice regularly and experiment with different ideas to improve your skills. Start small, gradually increase the complexity, and don’t be afraid to ask for help when you get stuck.
Conclusion
Building AI agents from scratch, especially using free and open-source tools, is an incredibly rewarding experience. This guide has provided you with a solid foundation, from setting up your development environment to building your first simple reflex agent. Remember that the journey doesn’t stop here. The world of AI agents is vast and constantly evolving.
Continue exploring different agent types, experimenting with new libraries and techniques, and tackling more complex projects. The possibilities are truly limitless, and the skills you develop will be invaluable in the years to come.
The ability to create intelligent systems is no longer a futuristic dream; it’s a skill you can cultivate today. By embracing the open-source community, leveraging free resources, and consistently practicing, you can unlock the full potential of AI agents. Whether you’re interested in building chatbots, game-playing AIs, or more specialized applications, the knowledge you’ve gained here is a powerful starting point.
Ready to take your AI agent development to the next level?
Vegavid Technology, a leading AI agent consulting company, can help you transform your AI agent ideas into reality. Our expert team offers tailored solutions for businesses of all sizes, from initial consultation and strategy development to custom AI agent design and deployment.
Contact Vegavid Technology today to discuss your project and discover how we can help you harness the power of intelligent agents.